In an era where data is at the heart of decision-making, automating workflows is no longer a luxury—it’s a necessity. Whether you’re a financial analyst, stock trader, or someone simply tracking the market, the idea of manually refreshing stock prices in spreadsheets can be both time-consuming and prone to error.
But what if you could automate the entire process, ensuring that your stock data is always up to date with just a few lines of code? Enter Python, the programming language that’s becoming increasingly essential for automating tasks and pulling data in real time.
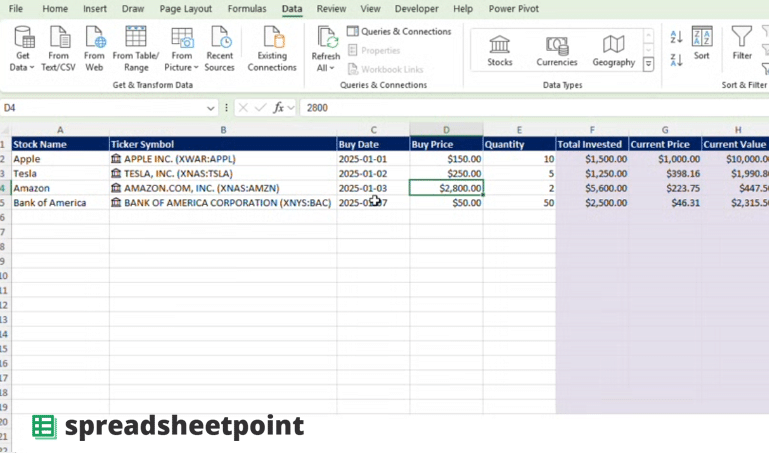
One of the most powerful applications of Python lies in its ability to interact with Google Sheets, a widely used tool for storing and analyzing data. By connecting Google Sheets to Python, users can automate the process of fetching live stock market data, ensuring that their financial records are updated with minimal manual intervention. This integration streamlines the workflow and creates opportunities for real-time analysis, making it an invaluable asset for anyone working in finance.
In this article, we’ll explore how Python can be paired with Google Sheets (or an Excel stock tracker) to automate live stock market data updates. We’ll break down the process step-by-step, from connecting your Python environment to Google Sheets, to fetching stock prices in real-time using the Yahoo Finance API, and finally, updating your Google Sheet with the latest market information.
Setting Up the Google Sheets API: A Step-by-Step Guide
Before we dive into the Python code, it’s important to understand how the connection between Python and Google Sheets works. This integration is made possible by the Google Sheets API, which allows external applications to interact with Google Sheets data securely. The connection requires authentication, ensuring that Python has the necessary permissions to update your sheets without compromising your data’s security. Not familiar with this language? There are many resources where you can learn Python.
Here’s how you can set up the connection between Python and Google Sheets:
- Create a Google Cloud Project: To use the Google Sheets API, you’ll first need to create a Google Cloud project. Navigate to the Google Cloud Console and create a new project. This project will serve as the foundation for managing your API keys and credentials.
- Enable the Google Sheets API: Once you have a project set up, the next step is to enable the Google Sheets API. This will allow Python to access your Google Sheets data. You can find this option in the API Library section of the Google Cloud Console.
- Create a Service Account: The next step is to create a service account within your project. This account will have the necessary permissions to access and edit your Google Sheets. During the creation process, you’ll generate a service account key, which is essentially a credential file used to authenticate Python’s access to the Google Sheets API.
- Share the Google Sheet with the Service Account: To give Python permission to access your Google Sheet, you need to share the sheet with the service account’s email address. This email address will be available in the credentials file you downloaded in the previous step. Once shared, the service account will be able to make changes to the sheet.
- Install the Required Python Libraries: To communicate with Google Sheets from Python, you’ll need to install a few libraries. Use pip to install
gspread
andoauth2client
—both of which help manage the Google Sheets API and the authentication process.
pip install gspread oauth2client
- Authenticate Python to Access Google Sheets: With the credentials file downloaded and the required libraries installed, the next step is to authenticate Python. You’ll load the credentials and authorize the Python script to access the Google Sheets API.
Fetching Live Stock Market Data with Yahoo Finance API
Now that Python has access to your Google Sheets, it’s time to set up the mechanism to fetch live stock market data. One of the most commonly used APIs for retrieving stock prices is Yahoo Finance, which provides real-time stock market data for a wide range of global markets.
You’ll use the yfinance
library, which is a convenient wrapper around the Yahoo Finance API. To install yfinance
, run:
pip install yfinance
With yfinance
installed, you can start fetching live stock prices. The following Python code snippet shows how to retrieve stock data for a specific ticker symbol (e.g., “AAPL” for Apple Inc.):
import yfinance as yf
# Fetch stock data for Apple
stock = yf.Ticker("AAPL")
stock_data = stock.history(period="1d")
# Display the latest closing price
print(stock_data['Close'].iloc[0])
This code pulls the most recent closing price for Apple’s stock and displays it. You can modify this to fetch data for other stocks or adjust the time frame (e.g., “1d” for daily, “1mo” for monthly, etc.).
Updating Google Sheets with Stock Market Data
The final step is to update your Google Sheets with the live stock market data you’ve fetched. To do this, you’ll need to insert the stock price into a specific cell of your Google Sheet. Here’s a simple example:
import gspread
from oauth2client.service_account import ServiceAccountCredentials
import yfinance as yf
# Authenticate and access the Google Sheet
scope = ["https://spreadsheets.google.com/feeds", "https://www.googleapis.com/auth/drive"]
credentials = ServiceAccountCredentials.from_json_keyfile_name('your-credentials-file.json', scope)
client = gspread.authorize(credentials)
# Open the Google Sheet by its name
sheet = client.open("Stock Prices").sheet1
# Fetch live stock data for Apple
stock = yf.Ticker("AAPL")
stock_data = stock.history(period="1d")
latest_price = stock_data['Close'].iloc[0]
# Update the Google Sheet with the latest stock price
sheet.update_cell(2, 2, latest_price)
This script authenticates Python, accesses the Google Sheet named “Stock Prices,” and updates the stock price of Apple in cell B2 with the most recent closing price.
Automating the Process
Once you have the basics in place, you can schedule this script to run at regular intervals using task schedulers like cron (Linux/macOS) or Task Scheduler (Windows). This will ensure that your stock prices are updated automatically without any manual intervention.
For instance, you could schedule the script to run every hour or once a day, depending on how often you want the data updated.
Not familiar with Python? Start with an online Python code editor to learn the basics.
By combining Python with Google Sheets, you can automate the process of fetching and updating live stock market data, saving time and ensuring accuracy. Whether you’re tracking multiple stocks or analyzing financial trends, this integration provides a powerful and efficient way to manage your data. The ability to automate such processes opens up a world of possibilities for real-time analysis, decision-making, and reporting.
As Python continues to gain traction in various industries, the potential for automating tasks and integrating with popular platforms like Google Sheets is virtually limitless. By mastering this integration, you can streamline your workflows and stay ahead in an increasingly data-driven world.
Related Reading: 30 Python Projects For Your Portfolio